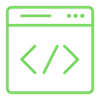
You may have asked yourself before how planes know the coordinates of their location on the map. In fact, planes know the coordinates of their location through the GPS module that is built into the plane control circuits, in this tutorial we will use one of those modules with Arduino. which is NEO-6M GPS module, which can accurately determine your location.
In this tutorial, we will learn about NEO-6M GPS module and how to interface it with Arduino to obtain GPS parameters such as latitude, longitude, altitude date, time, speed, and display them on your computer.
Connect the wires between the NEO-6M GPS module and the Arduino, as shown in the image below.
Connections from the NEO-6M GPS module to arduino :
• Vcc → Arduino 5V pin
• GND → Arduino GND pin
• RX PIN → Arduino pin 3
• TX PIN → Arduino pin 4
Now access the serial monitor on your Arduino IDE by clicking on the magnifying glass icon at the top right corner.
Now, as shown in the image below, the serial monitor displays the latitude, longitude, altitude date, time, speed, and display them on your computer.