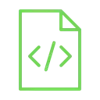
Sound is a very important aspect to consider in a number of projects and in this tutorial I will show you how we can make a simple sound dependent clap switch which can be used to control a number of devices for example lights in our home.
In this tutorial, you will learn how to create a sound-activated switch using an Arduino, a Microphone Module, and an LED. This project will enable you to control a light or other device with a simple clap.
Connect the wires between the Microphone Module and the Arduino and the LED as shown in the image below.
Connections from the Arduino to the breadboard:
• Arduino GND pin → Breadboard ground line
• Arduino 5V pin → Breadboard 5V line
Connections from the Microphone Module :
• Microphone Module VCC pin → Breadboard 5V line
• Microphone Module GND pin → Breadboard ground line
• Microphone Module A0 pin → Arduino pin A0
Connections from the LED :
• LED anode pin → 330 ohm resistor first pin
• LED cathode pin → Breadboard ground line
Connections from the 330 ohm resistor:
• 330 ohm resistor first pin → LED anode pin
• 330 ohm resistor second pin → Arduino pin 3
Once you’ve uploaded the code to the Arduino board, you will find that when you clap, the LED will toggle between on and off states. As shown in the video below.