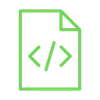
Controlling things remotely is a wonderful thing. Thanks to Arduino, you can control turning on and off lamps using an ir remote. You can also control the color of the lamp’s lighting.
In this tutorial, we will learn how to control an RGB LED to light up different colors by pressing different buttons on an IR remote.
Connect the wires between the ir sensor and the Arduino and the RGB led as shown in the image below.
Connections from the Arduino to the breadboard:
• Arduino GND pin → Breadboard ground line
• Arduino 5V pin → Breadboard 5V line
Connections from the RGB LED:
• Red led pin→220-ohm resistor→ Arduino pin 3
• Negative pin→ Breadboard ground line
• Green led pin→ 220-ohm resistor → Arduino pin 5
• Blue led pin→ 220-ohm resistor → Arduino pin 6
Connections from IR receiver to the Arduino:
• IR receiver GND pin (- pin) → Breadboard ground line
• IR receiver VCC pin (+ pin) → breadboard 5v line
• IR receiver signal pin → Arduino pin 9
Once you’ve uploaded the code to the Arduino board, you will find that you can control the RGB LED to light up different colors by pressing different buttons on the IR remote