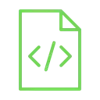
Smoke may often indicate that there is a fire in your home, so smoke detectors are very important in determining the occurrence of fires. Thanks to Arduino, you can build an accurate system to detect smoke levels.
In this tutorial, We will teach you how to build an accurate system to detect smoke levels using Arduino and the MQ135 gas sensor, so that it displays the smoke level on a crystal display, and when the gas percentage exceeds a certain value, an alarm sounds via a buzzer.
Connect the wires between the MQ-135 and the Arduino and the LCD and the buzzer as shown in the image below.
Connections from the Arduino to the breadboard:
• Arduino GND pin → Breadboard ground line
• Arduino 5V pin → Breadboard 5V line
Connection from the LCD with I2C Module:
• VCC pin → breadboard 5v line
• GND pin → breadboard GND line
• SDA pin → Arduino analog pin A4
• SCL pin → Arduino analog pin A5
Connections from the MQ-135 sensor:
• MQ-135 sensor VCC pin → Breadboard 5V line
• MQ-135 sensor GND pin → Breadboard ground line
• MQ-135 sensor A0 pin → Arduino pin A0
Connections from the Arduino to the passive buzzer:
• Arduino GND pin → buzzer GND pin (- pin)
• Arduino pin 3 → buzzer VCC pin (+ pin)
Once you've uploaded the code to the Arduino board, you will find that the LCD displays the smoke level and the buzzer emits an alarm sound when the smoke levels reach a certain value, as shown in the video below.