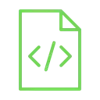
Metal detection is a great pastime that allows you to enjoy the outdoors, explore new places. A metal detector is a device used to detect the presence of metal in its proximity without physical contact. In this tutorial, we will build a metal detector using an Arduino.
In this tutorial, we will learn how to use an Arduino to create a metal detector that can detect the presence of metals, sound an alarm when metal is detected.
Connect the wires between the Arduino and the rest of the electronic components as shown in the image below.
Connections from the Arduino to the breadboard :
• Arduino GND pin → Breadboard ground line
• Arduino 5V pin → Breadboard 5V line
Connections from the 10 n capacitor :
• capacitor first pin → Breadboard ground line → coil first wire
• capacitor second pin → diode first pin → Arduino A1 pin
Connections from the diode :
• diode first pin → capacitor second pin → Arduino A1 pin
• diode second pin → 330 ohm resistor first pin
Connections from the 330 ohm resistor :
• 330 ohm resistor first pin → diode second pin
• 330 ohm resistor second pin → coil second wire → Arduino A0 pin
Connections from the passive buzzer :
• passive buzzer GND pin → Breadboard ground line
• passive buzzer VCC pin (+ pin) → Arduino pin 3
Once you’ve uploaded the code to the Arduino board, you will find that the buzzer will sound an alarm when metal is detected, as shown in the video below.