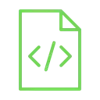
You may often need to control the movement of an object at a specific angle and speed. In this tutorial, we will use a joystick to control the servo motor shaft to sweep back and forth across 180 degrees with Arduino.
In this tutorial, we will use a joystick to control the servo motor shaft to sweep back and forth across 180 degrees with Arduino and display the angle of servo motor on a TFT Display.
Connect the wires between the TFT Display and the Arduino and the Servo motor and the joystick as shown in the image below.
Connections from the Arduino to the breadboard:
• Arduino GND pin → Breadboard ground line
• Arduino 5V pin → Breadboard 5V line
Connections from the servo motor:
• Servo GND pin→ Arduino GND pin
• Servo VCC pin→ Arduino VCC pin
• Servo signal pin → Arduino pin 4
Connections from the joystick:
• joystick GND pin→ Arduino GND pin
• joystick VCC pin→ Arduino VCC pin
• joystick x_axis pin → Arduino pin A0
Connections from the TFT Display to the breadboard:
• TFT Display VCC pin → Breadboard 5V line
• TFT Display GND pin → Breadboard ground line
• TFT Display LED pin → Breadboard 5V line
Connections from the l298n motor driver to arduino :
• TFT Display CS pin → Arduino pin 10
• TFT Display RST pin → Arduino pin 8
• TFT Display DC pin → Arduino pin 9
• TFT Display MOSI pin → Arduino pin 11
• TFT Display SCK pin → Arduino pin 13
• TFT Display MISO pin → Arduino pin 12
Once you’ve uploaded the code to the Arduino board, you will be able to use the joystick to control the servo motor shaft and the TFT Display will display the angle of the servo motor as shown in the video below: