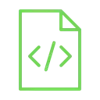
It matters a lot if your Arduino supports a phone chip. It may be used in a variety of applications, such as security systems to send alerts when anything goes wrong or to control devices from anywhere in the world via text messages. The SIM900 GSM module enables you to provide your Arduino with a SIM card to work like a cell phone!
In this tutorial we will control a small LED by sending a text message from your phone to the Arduino via the SIM900 GSM Shield.
Connect wires between the Arduino and the SIM900 GSM module as shown in the image below.
Connections from the Arduino to the breadboard:
• Arduino pin GND → Breadboard ground line
• Arduino pin 5V → Breadboard 5V line
Connections from the sim900 GSM module:
• TWO GND pin→ Breadboard ground line
• TXD pin → Arduino pin 7
• RXD pin → Arduino pin 8
Connections from the LED:
• Negative pin→ Breadboard ground line
• Positive pin→ Arduino pin 2
Now you must have correctly wired the SIM900 GSM module to the Arduino, as we explained in the wiring section, as well as uploaded the code to your Arduino board.
Try to send a message to the SIM card on your Arduino; if the message contains “ON,” whether in capital or small letters, you will notice that the LED is turned on.
If the message contains “OFF,” whether in capital or small letters, you will notice that the LED is turned off.
What do you think about the useful things that you can control remotely in this way?