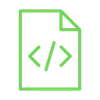
We are sure that you have seen an automatic door that opens and closes by itself. These kinds of gates are used in hotels, offices, and other places, and typically use special kinds of motors and sensors for their function. In this project, however, we will make an Automatic Gate opener using Arduino.
In this tutorial, we will teach you how to make your own automatic door opener using an ultrasonic sensor, servo motor, and Arduino.
Connect the wires between the TFT Display and the Arduino and the ultrasonic sensor and the servo motor as shown in the image below.
Connections from the Arduino to the breadboard:
• Arduino GND pin → Breadboard ground line
• Arduino 5V pin → Breadboard 5V line
Connections from the servo motor:
• Servo GND pin→ Arduino GND pin
• Servo VCC pin→ Arduino VCC pin
• Servo signal pin → Arduino pin 4
Connections from the ultrasonic sensor:
• ultrasonic sensor VCC pin → Breadboard 5V line
• ultrasonic sensor GND pin → Breadboard ground line
• ultrasonic sensor Trig pin → Arduino pin 3
• ultrasonic sensor Echo pin → Arduino pin 2
Connections from the TFT Display to the breadboard:
• TFT Display VCC pin → Breadboard 5V line
• TFT Display GND pin → Breadboard ground line
• TFT Display LED pin → Breadboard 5V line
Connections from the l298n motor driver to arduino :
• TFT Display CS pin → Arduino pin 10
• TFT Display RST pin → Arduino pin 8
• TFT Display DC pin → Arduino pin 9
• TFT Display MOSI pin → Arduino pin 11
• TFT Display SCK pin → Arduino pin 13
• TFT Display MISO pin → Arduino pin 12
Once you’ve uploaded the code to the Arduino board, you will find that the gate automatically opens when something passes in front of the ultrasonic sensor and displays a message on the TFT Display that says ‘object detected, gate will open’ as shown in the video below: