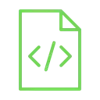
The HC-12 is a wireless communication module that is very useful, extremely powerful, and easy to use. With this module, you can have many applications like remote control, data logging, wireless programming, etc.
In this tutorial, we will use the HC-12 module to control a servo motor using a push button. You can use it to open and close doors, operate machinery, or even control valves.
The transmitter circuit wiring:
Connections from the Arduino to the breadboard:
• Arduino GND pin → Breadboard ground line
• Arduino 5V pin → Breadboard 5V line
Connections from the HC-12 module:
• GND pin→ Breadboard ground line
• VCC pin→ Breadboard 5V line
• TXD pin → Arduino pin 7
• RXD pin → Arduino pin 8
Connections from the first push button:
• First pin→ Breadboard 5v line
• Second pin→ Arduino pin 2
• Second pin→10KΩ resistor → Breadboard Ground line
Connections from the second push button:
• First pin→ Breadboard 5v line
• Second pin→ Arduino pin 5
• Second pin→10KΩ resistor → Breadboard Ground line
The receiver circuit wiring:
Connections from the Arduino to the breadboard:
• Arduino pin GND → Breadboard ground line
• Arduino pin 5V → Breadboard 5V line
Connections from the HC-12 module:
• GND pin→ Breadboard ground line
• VCC pin→ Breadboard 5V line
• TXD pin → Arduino pin 7
• RXD pin → Arduino pin 8
Connections from the servo motor:
• GND pin→ Breadboard ground line
• VCC pin→ Breadboard 5V line
• Signal pin → Arduino pin 9
The transmitter circuit code:
The reciver circuit code:
When you press the first push button, you will observe the servo motor moving at 90 degrees to open the door, but when you press the second push button, you will observe the servo motor going back to its origin again!