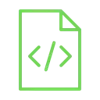
To control a DC motor with an Arduino, it’s important to know that you will need an intermediary between the Arduino and the motor, such as a motor driver. In this tutorial, we’ll learn how to use the popular motor driver L298N, which is commonly used for controlling DC motors.
In this tutorial, we will learn how to use the L298N motor driver with the Arduino, and we will program the Arduino to turn the motor on and off, as well as change its direction and speed of rotation. We will program the Arduino to do this without using any external control accessories.
Connect the wires between the L298N motor driver and the Arduino as shown in the image below.
Connections from the l298n motor driver to arduino :
• ENABLE A pin→ Arduino pin 10
• Input1 pin→ Arduino pin 9
• Input2 pin→ Arduino pin 8
• Input3 pin→ Arduino pin 7
• Input4 pin→ Arduino pin 6
• ENABLE B pin→ Arduino pin 5
Connections from 18650 battery holder to the l298n motor driver:
• positive wire of the 18650 battery holder → VS terminal
• negative wire of the 18650 battery holder → Gnd terminal + Arduino GND pin
Connections from the motor 1 to the l298n motor driver:
• Motor 1 first wire → Out1
• Motor 1 second wire → Out2
Connections from the motor 2 to the l298n motor driver:
• Motor 2 first wire → Out3
• Motor 2 second wire → Out4
Once you’ve uploaded the code to the Arduino board, In the first three seconds, you will find that the first motor is spinning while the other is stopped, as shown on the right GIF:
While in the next three seconds, the second motor will spin, while the first will stop:
Then the two motors will rotate together in the same direction for three seconds at a speed specified in the code(PWM=150):
Then the two motors will rotate together in the opposite direction for a period of three seconds at a speed faster than the previous stage. It was specified in the code to be the maximum speed (PWM = 255):