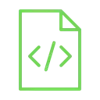
Using Arduino, you can read a file from an SD card and extract variables from it! This can be very useful if you want to store data on an SD card, such as sensor readings or GPS data. Then process this information or use it in another system.
In this tutorial, we will use the Arduino to open and read a file from a memory card. Then process it from the string state to extract the variables.
Connect the wires between the SD card reader and the Arduino, as shown in the image below.
Connections from the Arduino SD card module:
• Arduino GND pin → SD card module GND pin (- pin)
• Arduino 5V pin → SD card module VCC pin (+pin)
• Arduino pin 12 → SD card module MISO pin
• Arduino pin 11 → SD card module MOSI pin
• Arduino pin 13 → SD card module SCK pin
• Arduino pin 4 → SD card module CS pin
Now access the serial monitor on your Arduino IDE by clicking on the magnifying glass icon at the top right corner.
Now, as we see in the following image, the serial monitor prints the TXT file line by line, and after each line, the code extracts the temperature and humidity values from it. We can use these values in more advanced operations, like making an actuator perform a specific task.
If you remove your SD card while the code is running, you will get an error message with the status of the SD card through the serial monitor, getting feedback during the process is very useful and makes it easier for you to discover errors without looking for more than one reason.