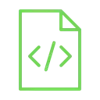
Have you ever seen a smart lock in which you have to put the card on the reader to get access? Well, with Arduino, we can create our own smart lock using an RFID lock system that requires a unique card to perform a specific action. In our case, we will use it to open a door with a servo motor.
In this tutorial, we will make an RFID lock system that opens a door when a unique card is used with the device, and we will use a servo motor to open and close that door.
Connect the wires between the RFID module and the Arduino and the servo and the buzzer as shown in the image below.
Connections from the Arduino to the breadboard:
• Arduino GND pin →Breadboard ground line
• Arduino 5V pin →Breadboard 5V line
Connections fromthe RFID reader to the Arduino:
• RFID 3.3V pin →Arduino VCC (3.3V) pin
• RFID RST pin →Arduino pin 9
• RFID GND pin →Breadboard ground line
• RFID IRQ pin →unconnected
• RFID MISO pin →Arduino pin 12
• RFID MOSI pin →Arduino pin 11
• RFID SCK pin →Arduino pin 13
• RFID SDA pin →Arduino pin 10
Connections fromthe servo motor:
• Servo GND pin→breadboard GND line
• Servo 5V pin→breadboard 5V line
• Servo signal pin→ Arduino pin 3
Connections fromthe passive buzzer :
• passive buzzerGND pin → Breadboard ground line
• passive buzzerVCC pin (+ pin) → Arduino pin 6
Connections fromthe Green LED :
• Green LED anodepin → Arduino pin 5
• Green LED cathodepin → 330 ohm resistor first pin → Breadboard ground line
Connections fromthe Red LED :
• Red LED anode pin→ Arduino pin 7
• Red LED cathodepin → 330 ohm resistor first pin → Breadboard ground line
Once you’ve uploaded the code to the Arduino board, you will find that the servo will rotate to open the door when a unique card is brought near the RFID module, then the door will close automatically after a delay of three seconds.