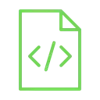
Imagine being able to stay relaxed and secure indoors while still keeping an eye on the weather conditions outside. With the HC-12 sensor, you can wirelessly transmit and receive temperature and humidity readings without ever having to step outside!
In this tutorial, we will learn how to use an HC-12 module and an Arduino to send temperature and humidity data from a DHT11 sensor, and then receive the data using another Arduino and HC-12 module. The HC-12 can transmit and receive data from distances up to 1 kilometer.
The transmitter wiring:
Follow the wiring diagram shown in the image below to connect the DHT11 sensor and the HC-12 module to the Arduino.
Connections from the Arduino to the breadboard:
• Arduino 5v pin → breadboard 5v line
• Arduino GND pin → breadboard GND line
Connection form the DHT11:
• GND pin → breadboard GND line
• Signal pin → Arduino digital pin 2
• VCC pin → breadboard 5v line
Connection from the HC 12 module:
• VCC pin → breadboard 5v line
• GND pin → breadboard GND line
• TX pin → Arduino pin 3
• RX pin → Arduino pin 4
The receiver wiring:
Follow the wiring diagram shown in the image below to connect the HC-12 module and the LCD to the Arduino.
Connections from the Arduino to the breadboard:
• Arduino 5v pin → breadboard 5v line
• Arduino GND pin → breadboard GND line
Connection from the HC 12 module:
• VCC pin → breadboard 5v line
• GND pin → breadboard GND line
• TX pin → Arduino pin 10
• RX pin → Arduino pin 11
Connections from the LCD:
• VCC pin → breadboard 5v line
• GND pin→ breadboard GND line
• SDA pin→ Arduino analog pin A4
• SCL pin → Arduino analog pin A5
The Transmitter code:
The code function is to read the temperature and humidity values from a DHT11 sensor and transmitting them wirelessly via an HC-12 module.
The receiver code:
The code function is to receive the temperature and humidity data from the transmitter module via the HC12 wireless module and displays it on an LCD.
Once you’ve wired the two circuits and uploaded the code, put the transmitter circuit outdoors to monitor the weather status.
The readings from the DHT11 will be transmitted through the HC-12 wireless module and displayed on the LCD by the receiver circuit. The readings will update every one second because of the delay that we have added to the code.