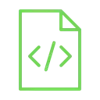
يُعد تخطيط إشارات القلب أحد الأدوات المهمة في مجال الرعاية الصحية، حيث يوفر معلومات دقيقة عن نشاط القلب وحالته الكهربائية. في هذا المشروع، سنقوم ببناء نظام يعتمد على لوحة أردوينو لرسم مخطط إشارات القلب (ECG).
في هذا المشروع، سنقوم بتطوير نظام متكامل لرسم مخطط إشارات القلب (ECG) باستخدام لوحة أردوينو ومستشعر مخصص لالتقاط الإشارات الحيوية. في الجزء الأول، سيتم عرض القراءات مباشرة على شاشة، مع تمثيل البيانات بيانياً على الكمبيوتر لتوفير رؤية أوضح لحالة القلب. بالإضافة إلى ذلك، في الجزء الثاني، سنقوم بعرض الرسم البياني لإشارات القلب على شاشة OLED.
قم بتوصيل الأقطاب الخاصة بالحساس في أماكن النقاط كما في الصورة رقم (1) أو كما في الصورة رقم (2).
قم بتوصيل الأسلاك بين لوحة الأردوينو وحساس إشارة القلب وشاشة الOLED كما في الصورة التالية:
التوصيلات من الاردوينو الى لوحة التجارب :
• منفذ ال 5 فولت ← المنافذ الموجبة بلوحة التجارب
• منفذ الجراوند ← المنافذ السالبة بلوحة التجارب
التوصيلات من حساس رسم اشارات القلب الECG Sensor :
• المنفذ الGND للحساس ← المنافذ السالبة بلوحة التجارب
• منفذ 3.3v للحساس ← منفذ الطاقة 3.3v فى لوحة الاردوينو
• المنفذ OUTPUT للحساس← منفذ رقم A0فى لوحة الاردوينو
• المنفذ LO- للحساس ← منفذ رقم 11 فى لوحة الاردوينو
• المنفذ LO+ للحساس ← منفذ رقم 10 فى لوحة الاردوينو
التوصيلات من الشاشة الOLED :
• منفذ ال VCC للشاشة ← المنافذ الموجبة بلوحة التجارب
• منفذ ال GND للشاشة ← المنافذ السالبة بلوحة التجارب
• منفذ SDA للشاشة ← منفذ رقم A4 فى لوحة الاردوينو
• منفذ SCL للشاشة ← منفذ رقم A5 فى لوحة الاردوينو
بعد رفع الكود البرمجي الأول، وتركيب أقطاب حساس الـ ECG، قم بفتح الـ Serial Plotter ستجد أنه يتم رسم مخطط إشارات القلب على الشاشة، وإذا قمت برفع الكود البرمجي الثاني على لوحة الأردوينو ستجد أنه يتم عرض منحنى رسم بياني لمخطط إشارات القلب على شاشة الـ OLED المتصلة بالأردوينو.