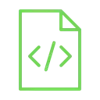
The HX711 IC’s Load Cell Amplifier is a compact breakout board that makes it simple to read load cells for weight measurement. You can read changes in the load cell’s resistance by connecting the amplifier to your microcontroller ( in this article, we are using Arduino), and with a little calibration, you can get pretty precise weight readings.
In this tutorial, we will make a simple weighing scale using Arduino UNO & HX711 Sensor. The electronic weighing device uses a load cell to measure the weight produced by the load, First, we will calibrate the load cell, and then use the cell as a digital scale.
Connect the wires between the HX711 and the load cell and the Arduino as shown in the image below:
Connections from the load cell to the HX711 :
• load cell red wire → HX711 pin E+
• load cell black wire → HX711 pin E-
• load cell white wire → HX711 pin A-
• load cell green wire → HX711 pin A+
Connections from the HX711 to the Arduino :
• VCC → Arduino pin 3.3 volt
• DT → Arduino pin 2
• SCK → Arduino pin 3
• GND → Arduino pin Gnd
There are two programs; one is the calibration program (finding the calibration factor). Another code is weight measurement program, the calibration factor found from the calibration program code need to be entered in weight measurement program.
The calibration factor determines the accuracy of the weight measurement.
Calibrations Program code:
now access the serial monitor on your Arduino IDE by clicking on the magnifying glass icon at the top right corner.
Now, as shown in the image below, the serial monitor displays the result of calibration on your computer.
In my case, during the calibration process, I used a known weight of 1 kilo, you can use any known weight you have.
calibration factor will be the (reading)/(known weight).
calibration factor = -229924/1000 = -229.924
Now we put the calibration factor in the next code.
Now access the serial monitor on your Arduino IDE by clicking on the magnifying glass icon at the top right corner.
Now, as shown in the image below, the serial monitor displays the weight on your computer.