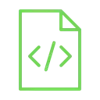
There are many different kinds of motors, each of which has a variety of uses. One type is the stepper motor, which got its name because it moves in precise steps and allows you to control the movement speed and direction with high precision.
In this tutorial, we will use the Raspberry pi 5 and motor driver to control the stepper motor. We will make it rotates a complete cycle in the right direction and then rotates a complete cycle in the opposite direction.
Connect the wires between the stepper motor driver module and the Raspberry pi 5 as shown in the image below.
Connections from the stepper motor driver module:
• Stepper motor driver module GND pin→ Raspberry pi 5 GPIO GND pin
• Stepper motor driver module VCC pin→ Raspberry pi 5 GPIO 5V pin
• Stepper motor driver module IN1 pin → Raspberry pi 5 GPIO pin 14
• Stepper motor driver module IN2 pin → Raspberry pi 5 GPIO pin 15
• Stepper motor driver module IN3 pin → Raspberry pi 5 GPIO pin 18
• Stepper motor driver module IN4 pin → Raspberry pi 5 GPIO pin 23
Now on your Raspberry Pi, click on the menu, then choose programming, then open the Thonny ide program.
Now copy that code into it, The function of this code is to make the Raspberry Pi 5 board controls the movement of the stepper motor so that it rotates a complete cycle in the right direction and then rotates a complete cycle in the opposite direction.
Now run the code, you will find that the Raspberry Pi 5 board controls the movement of the stepper motor so that it rotates a complete cycle in the right direction and then rotates a complete cycle in the opposite direction.