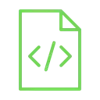
The security system that we will make in this tutorial is a complete wireless security solution that includes a PIR sensor, GSM module and a buzzer. The PIR sensor detects motion and sends an alert through a text message to your mobile phone using the SIM900 GSM module.
In this tutorial, we will use the PIR sensor with Arduino and the SIM900 GSM shield to send a message to your phone that alerts you when someone enters your house!
Connect the wires between the SIM900 GSM shield and the Arduino and the PIR sensor and the the buzzer as shown in the image below.
Connections from the Arduino to the breadboard:
• Arduino GND pin → Breadboard ground line
• Arduino 5V pin → Breadboard 5V line
Connections from the sim900 GSM module:
• TWO GND pin→ Breadboard ground line
• TXD pin → Arduino pin 7
• RXD pin → Arduino pin 8
Connections from the PIR sensor:
• positive pin→ Breadboard 5V line
• Signal pin → Arduino pin 2
• Negative pin → Breadboard ground line
Connections from the buzzer:
• Negative pin→ Breadboard ground line
• Positive pin→ Arduino pin 11
To test the PIR sensor, try to move in front of it, the sensor will be triggered and the buzzer will sound an alert. You will also receive an SMS on your phone number alerting you that there is someone in your house.