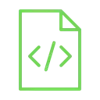
A memory card is a small chip that allows us to store data. It comes with different storage capacities and is used in many applications in our daily lives, in smartphones, and cameras.
In this tutorial we will get the temperature and humidity values from the DHT11 sensor. Then we will use the SD card module to save the values in a TXT file. We will also draw a graph of the temperature and humidity through the Excel program, and we will learn how to display time and date using Arduino.
Connect the wires between the soil moisture sensor and the Arduino, as shown in the image below.
Connections from the Arduino to the breadboard:
• Arduino GND pin → Breadboard ground line
• Arduino 5V pin → Breadboard 5V line
Connections from the DHT11:
• positive pin→ Breadboard 5V line
• Out pin → Arduino pin 3
• Negative pin → Breadboard ground line
Connections from the SD card module:
• GND pin → Breadboard ground line
• VCC pin → Breadboard 5V line
• MISO pin→ 12
• MOSI pin→ 11
• SCK pin → 13
• CS pin → Arduino pin 4
Now access the serial monitor on your Arduino IDE by clicking on the magnifying glass icon at the top right corner.
Now, as we see in the following image, the serial monitor displays the current time and date, along with temperature and humidity values. The value is updated and
printed every 30 seconds because of the delay we added in our code.
To obtain some values, you can wait for a period of time, like 30 minutes, and then connect the micro-SD card to your computer.
You will find a TXT file on it with the name we specified on the code, LOGGERCD.txt. When you open it, you will see the same data we have printed through the serial
monitor.
If you remove your SD card while the code is running, you will get an error message with the status of the SD card through the serial monitor.
We can import the TXT file into Excel to create a chart between time and temperature and also between time and humidity. This will be very useful to see and
understand what really happens to temperature and humidity over time, We can also recognize the relationship between temperature and humidity, which is an
inversely proportional relationship.
If you have data for several hours or days, it will be quite useful since you may use it to do some analysis, such as finding the warmest day or the average daily
temperature.