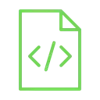
There are many different kinds of motors, each of which has a variety of uses. One type is the stepper motor, which got its name because it moves in precise steps and allows you to control the movement speed and direction with high precision. This type of motor is used in many devices that you may use in your daily life, such as 3D scanners, 3D printers, and even robots.
In this tutorial we will use the Arduino and motor driver to control the stepper motor. We will make it move with a specific direction and acceleration.
Connect the wires between the stepper motor and the Arduino, as shown in the image below.
Connections from the motor driver to the Arduino:
• Motor driver GND pin (- pin) → Arduino GND pin
• Motor driver VCC pin (+ pin) → Arduino 5V pin
• Motor driver IN1 pin → Arduino pin 11
• Motor driver IN2 pin → Arduino pin 10
• Motor driver IN3 pin → Arduino pin 9
• Motor driver IN4 pin → Arduino pin 8
After uploading the code to your Arduino board, you will observe the stepper motor rotating slowly and making nine revolutions before changing direction and repeating the action. The driver LEDs will also flash in response to the signals transmitted from the driver to the motor.
You can change the number of revolutions, acceleration and speed in the code with the specific value that you need.