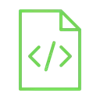
The RFID reader is a module that reads a unique number from a tag or a card when it comes near it. You can then use the scanned number to identify the card holder.
In this tutorial, we will use the RFID reader to read the data from the tag and then print a message on your computer. We can use this project to open a gate so that only the person with the correct information on his tag can enter.
To set up the RFID reader in the correct way, follow the instructions below. The image demonstrates how to connect the wires between the RFID reader and the Arduino. Once the RFID reader and the Arduino are connected to each other, connect the Arduino to your computer using the USB cable.
Connections from the RFID reader to the Arduino:
• RFID 3.3V pin → Arduino VCC (3.3V) pin
• RFID RST pin → Arduino pin 9
• RFID GND pin → Arduino GND pin
• RFID IRQ pin → unconnected
• RFID MISO pin → Arduino pin 12
• RFID MOSI pin → Arduino pin 11
• RFID SCK pin → Arduino pin 13
• RFID SDA pin → Arduino pin 10
Now access the serial monitor on your Arduino IDE by clicking on the magnifying glass icon at the top right corner.
Now, as we see in the following image, the serial monitor will print a message that asks you to approximate the tag from the RFID.
When you scan the correct tag with the RFID reader, a message with the allowed access will be printed.
If you use another different tag that is not defined in the code, it will print an access denied message.